PROJECT: PlanWithEase
Hi! I am Yunwei, a student from the National University of Singapore (NUS). I love to build applications that improve people’s lives.
This portfolio documents my involvement in a software development project, through which my teammates and me created a degree planner application within one school semester. This portfolio shows what the project is about and my contribution to the project.
Overview
For this project, we aim to simplify the process of degree-planning among NUS information security students.
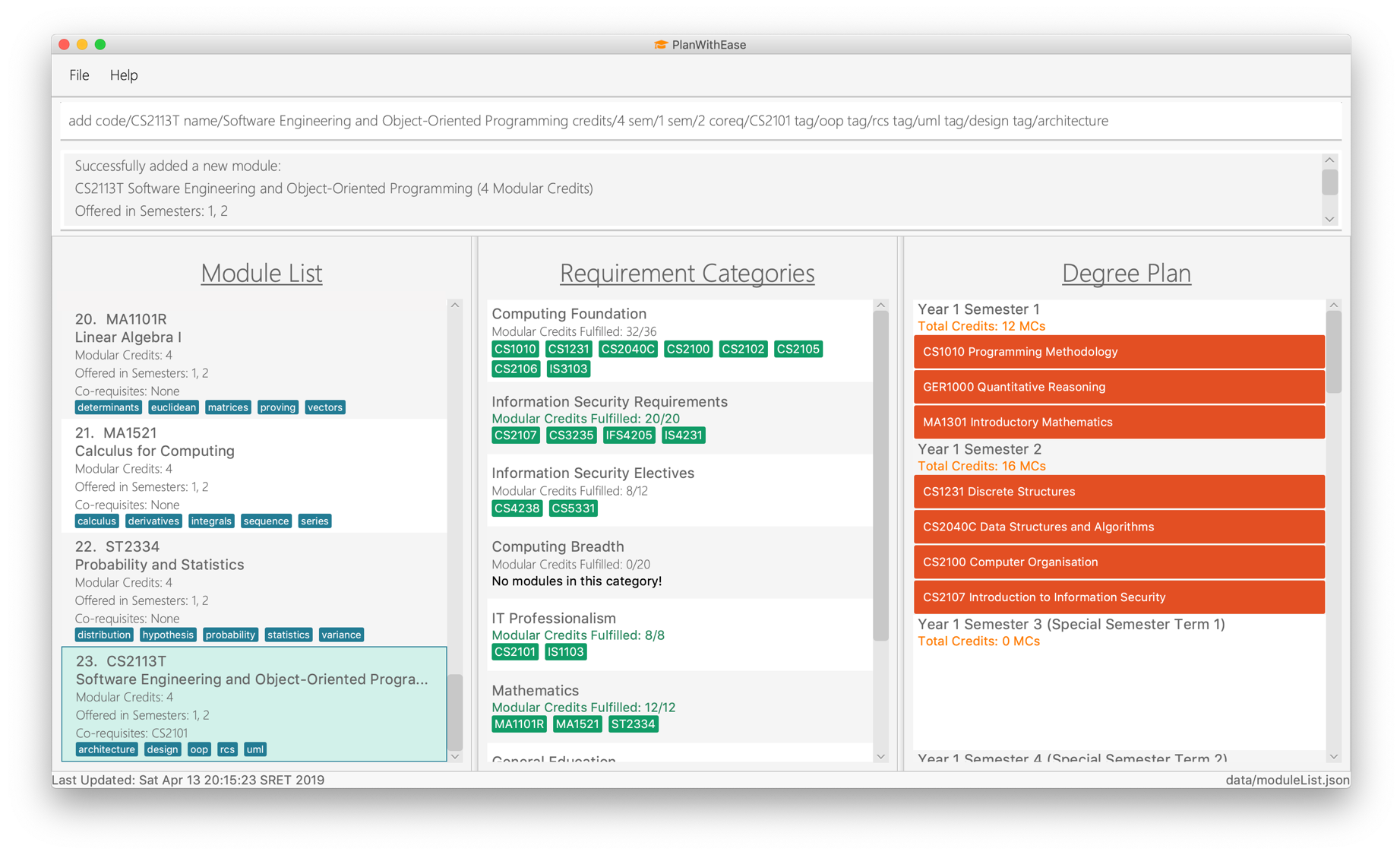
As a developer, my role in the project was writing codes to enable users to:
-
Add modules to the degree plan.
-
Remove modules from the degree plan.
-
Know the modules suggested to put inside the degree plan.
Summary of contributions
This section summarises my contribution to the project. |
-
Major enhancement: added the ability to add/remove module codes to/from the degree plan
-
What it does: allows the user to add module codes to the degree plan, and remove the codes from the degree plan.
-
Justification: This feature can be crucial because the degree plan is an important section that enables the users to plan well. Therefore, adding and removing the module codes are essential features that make PWE fulfill its purpose.
-
Highlights: This enhancement aims to prevent possible errors, including the hidden ones that may occur when other parts of the software get modified. Besides, the model and logic implementation for the feature is kept as minimalistic as possible to reduce unnecessary coupling.
-
-
Minor enhancement: added a command to suggest modules to put inside the degree plan.
-
Code contributed: [Commits] [RepoSense Code Contribution Dashboard]
-
Project management:
-
Assigned and managed "issues" for "planner_add", "planner_remove" and "planner_suggest" commands.
-
PR reviewed: https://github.com/CS2113-AY1819S2-T09-1/main/pull/146
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users to facilitate mastering of PlanWithEase's features. . |
Adding modules : planner_add

Want to add modules to the degree plan?
Make use of the planner_add
command to add one or multiple modules to the degree plan.
Command Format: planner_add year/YEAR sem/SEMESTER code/CODE [code/CODE]…
|
Examples:
-
planner_add year/1 sem/3 code/CS1010
Adds moduleCS1010
to year 1 semester 3 (special term semester 1) of the degree plan. -
planner_add year/1 sem/4 code/CS1231 code/CS2040C
Adds modulesCS1231
andCS2040C
to year 1 semester 4 (special term semester 2) of the degree plan. -
planner_add year/1 sem/2 code/CS2113T code/CS2107
Adds modulesCS2113T
andCS2107
to year 1 semester 2 of the degree plan.
Removing modules : planner_remove

Want to remove modules from the degree plan?
Make use of planner_remove
command to remove one or multiple modules from the degree plan.
Command Format: planner_remove code/CODE [code/CODE]…
|
Examples:
-
planner_remove code/CS1231
Removes moduleCS1231
from the degree plan. -
planner_remove code/CS2100 code/CS2040C
Removes modulesCS2100
andCS2040C
from the degree plan. ==== Using degree plan to suggest modules :planner_suggest
Having trouble identifying modules to add to the degree plan?
Make use of planner_suggest
command to find out recommended modules to add to the degree plan.
Command Format: planner_suggest credits/CREDITS [tag/TAG]…
When this command is successfully executed, the result box will display modules recommended.
|
Examples:
-
planner_suggest credits/4
Suggests maximum 10 modules not found in the degree plan, prioritizing modules with credits closer to 4. If tie, prioritizes according to alphabetical order. -
planner_suggest credits/2 tag/algorithms
Suggests maximum 10 modules not found in the degree plan, prioritizing modules with tagalgorithms
. If tie, prioritizes according to credits closer to 2. If tie again, prioritizes according to alphabetical order.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Planner Add Feature
The planner_add
command in PWE is used to add module code(s) to the degree plan.
Current implementation
The planner_add
command requires the PlannerAddCommandParser
class to parse the user input provided. The
parsed data will then be passed to the PlannerAddCommand
class.
The input should contain the year and semester of the degree plan as well as the module code(s) to be added.
PlannerAddCommandParser
will throw an error if the user input does not match the command format.
When PlannerAddCommand
receives the parsed data, it will perform the following checks:
-
Check if the year and semester exist in the degree plan
-
Check if the module codes provided exist in PWE through
model.hasModuleCode
-
Check if the module codes have already been added to the degree plan
-
Check if the co-requisites of modules provided already exist in other semesters of the degree plan
PlannerAddCommand
will throw an error if any of the above checks fails.
After passing all of the above checks, PlannerAddCommand
updates the context in ModelManager
through
setDegreePlanner
.
In addition to adding module code(s) to the degree plan, the PlannerAddCommand
class also saves the
current database state through commitApplication
(for undo/redo functions).
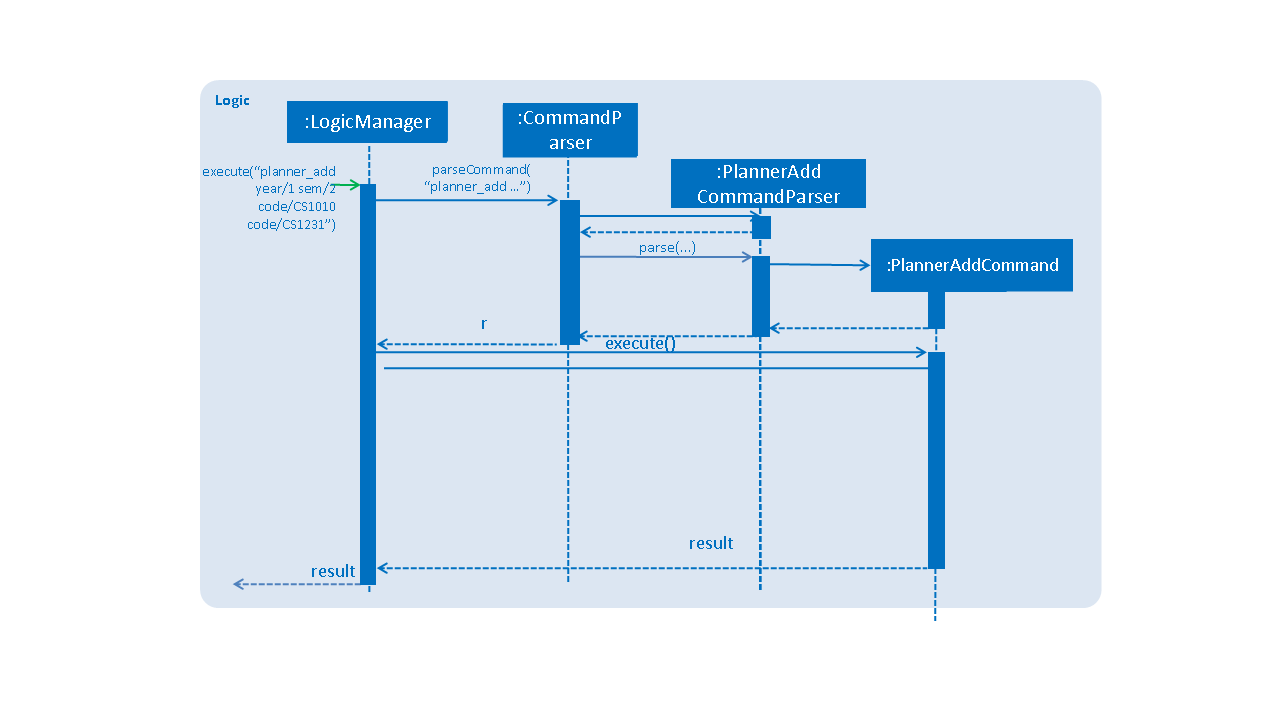
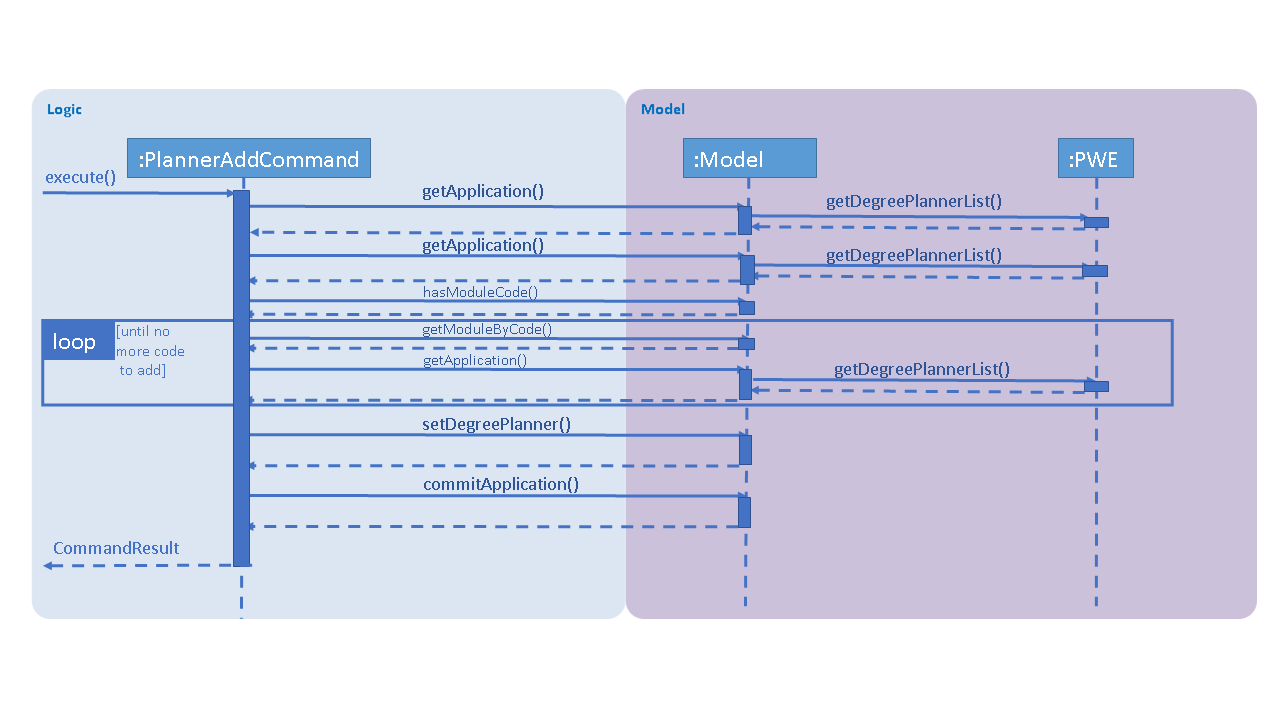
Design Considerations
Aspect: Choice of whether to deal with certain possible errors when the application gets modified.
-
Alternative 1 (current choice): Checking for invalid co-requisites as well as non-existent year and semester in degree plan.
Pros |
Software is more secure against possible errors. |
Cons |
Harder to maintain. |
-
Alternative 2: Skipping checks for invalid co-requisites as well as non-existent year and semester in degree plan.
Pros |
Easier to maintain. |
Cons |
When certain parts of the software get modified, some errors may occur with this command. For instance, a user may use |
Planner Remove Feature
The planner_remove
command in PWE is used to remove module code(s) from the degree plan.
Current implementation
The planner_remove
command requires the PlannerRemoveCommandParser
class to parse the user input provided. The
parsed data will then be passed to the PlannerRemoveCommand
class.
The input should contain the module code(s) to be removed.
PlannerRemoveCommandParser
will throw an error if the user input does not match the command format.
When PlannerRemoveCommand
receives the parsed data, it will perform the following checks:
-
Check if the module codes to remove exist in the degree plan.
PlannerRemoveCommand
will throw an error if any of the above checks fails.
After passing all of the above checks, PlannerRemoveCommand
updates the context in ModelManager
through
setDegreePlanner
.
In addition to removing module code(s) from the degree plan, the PlannerRemoveCommand
class also saves the
current database state through commitApplication
(for undo/redo functions).
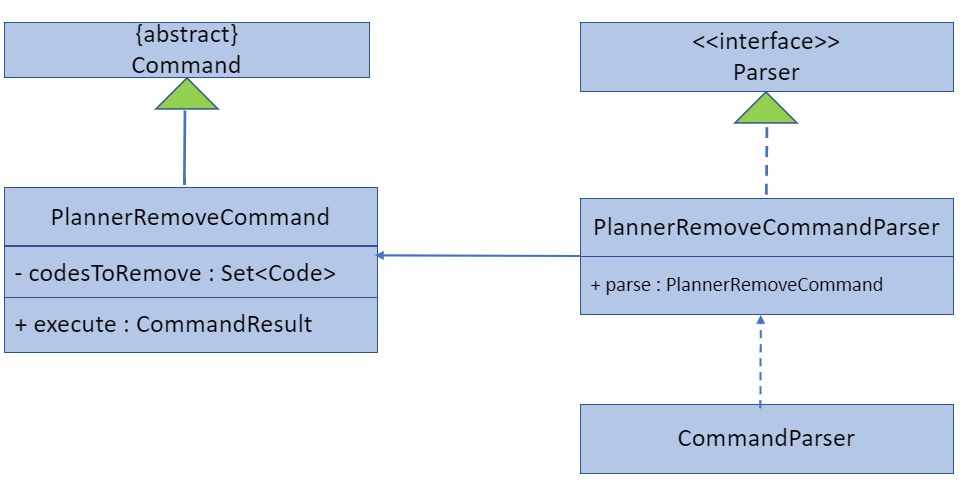
Design Considerations
Aspect: Choice of whether to use getDegreePlannerByCode
method for removing module codes.
-
Alternative 1 (current choice): Looping through all semesters of the degree plan instead of using
getDegreePlannerByCode
to identify the relevant semesters of the codes to remove.
Pros |
Simpler code. |
Cons |
May loop through extra semesters when there are few module codes to remove. |
-
Alternative 2: Using
getDegreePlannerByCode
method to identify the relevant semesters containing the code to remove. Removing the codes in the selected semester only.
Pros |
When there are few module codes to remove, the method may incur in slightly less overhead. |
Cons |
When there are N modules to remove, while the current method only needs 16 (total number of semesters) outer loops,
this alternative method needs N outer loops. Moreover, |
Planner Suggest Feature
The planner_suggest
command in PWE is used to suggest module code(s) to add to the degree plan.
Current implementation
The planner_suggest
command requires the PlannerSuggestCommandParser
class to parse the user input provided. The
parsed data will then be passed to the PlannerSuggestCommand
class.
The input should contain the desirable credits. Another optional input is the desirable tags.
PlannerSuggestCommandParser
will throw an error if the user input does not match the command format.
After passing the above check, PlannerSuggestCommand
will make the result box display 3 lists of modules. The first list
is the main recommendation list that sorts the modules in a specific way and displays maximum 10 most recommended modules.
The second and the third list respectively displays the modules with matching tags and modules with matching credits. The
modules in the two lists should also exist in the main recommendation list.
If tag
is supplied as a parameter in input, modules will be sorted according to tags first. Modules with greater number
of tags that match the desirable tags will be prioritized. If tie, modules with credits closer to the desirable credits
will be prioritized. If tie again, modules will be sorted according to alphabetical order.
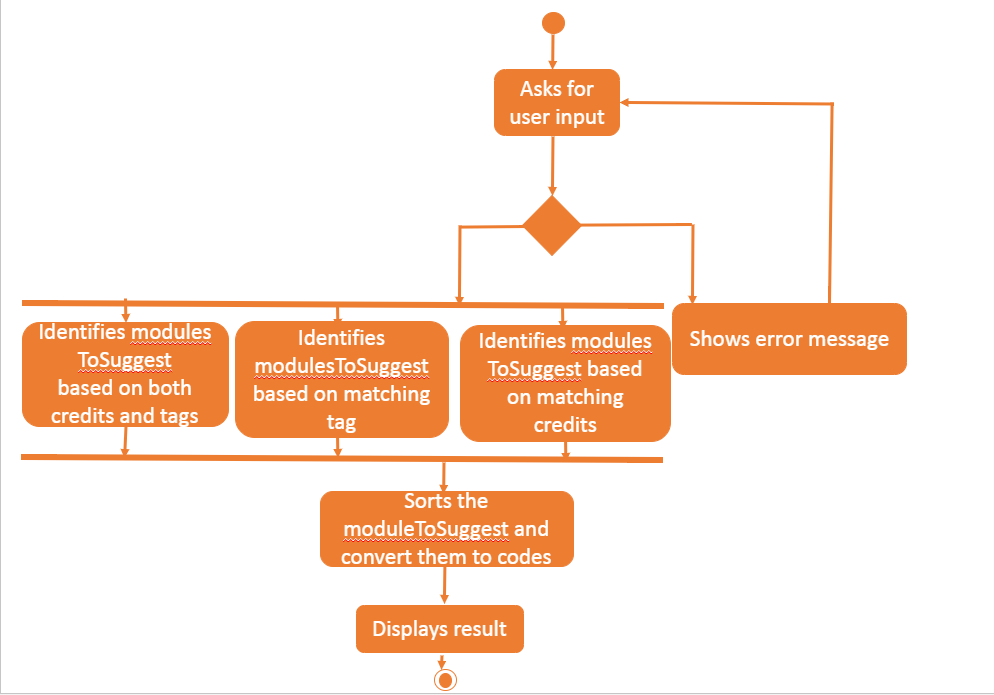
Design Considerations
Aspect: Choice of where to put ModuleToSuggest
class.
-
Alternative 1 (current choice): Put
ModuleToSuggest
as inner class ofPlannerSuggestCommand
class.
Pros |
Cleaner model for the Application. |
Cons |
The inner class has access to the private and protected members of the outer class, which can be unnecessary. |
-
Alternative 2: Put
ModuleToSuggest
as a separate class apart fromPlannerSuggestCommand
class.
Pros |
Better encapsulation for |
Cons |
As no other class needs to access the |